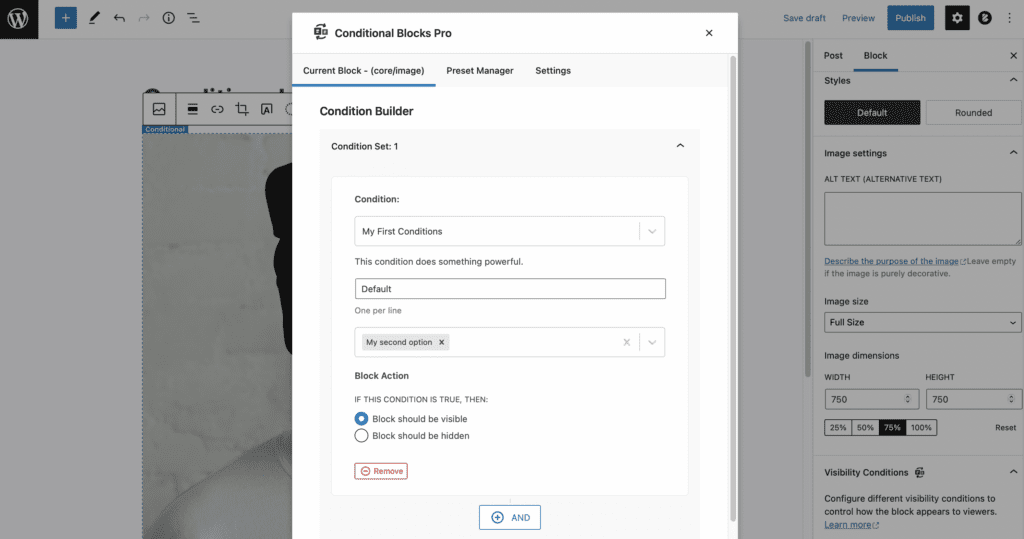
This documentation is a guide to create and register custom visibility condition within the Conditional Blocks WordPress plugin.
Custom conditions are created using what we call the Conditions API (v1).
We’ve prepared a documentation to explain the terminology used within Conditional Blocks to help new comers.
Benefits of Custom Conditions
- Create completely new and unique conditions
- Use your own conditions in combination with existing features, Conditions Sets for AND, OR logic.
- Native integration with the Condition Builder.
- Full support with existing conditions (AND, OR logic & presets).
- Use multiple field types to configure parameters for each Condition Check.
- The Conditions API is available in both Conditional Block (Free & Pro).
Each custom condition is programmatically translated from PHP into JavaScript (React), and then added to the WordPress Editor.
Who should use the Conditions API?
The Conditions API is designed to be used by developers, agencies and hobbyists to create powerful integration between the WordPress Block Editor and any third-party plugins.
We are committed to keep the Conditions API seamless & up to date in all future version of the Conditional Blocks Plugin.
How to create custom conditions
Creating your own custom visibility conditions to use with WordPress can be done using a few steps.
This guide will show you how to register new data within the Conditional Blocks plugin.
Register a Condition Category
You can create your own category for conditions within the Condition Builder. The purpose of each category make it easier for users to find related types of conditions.
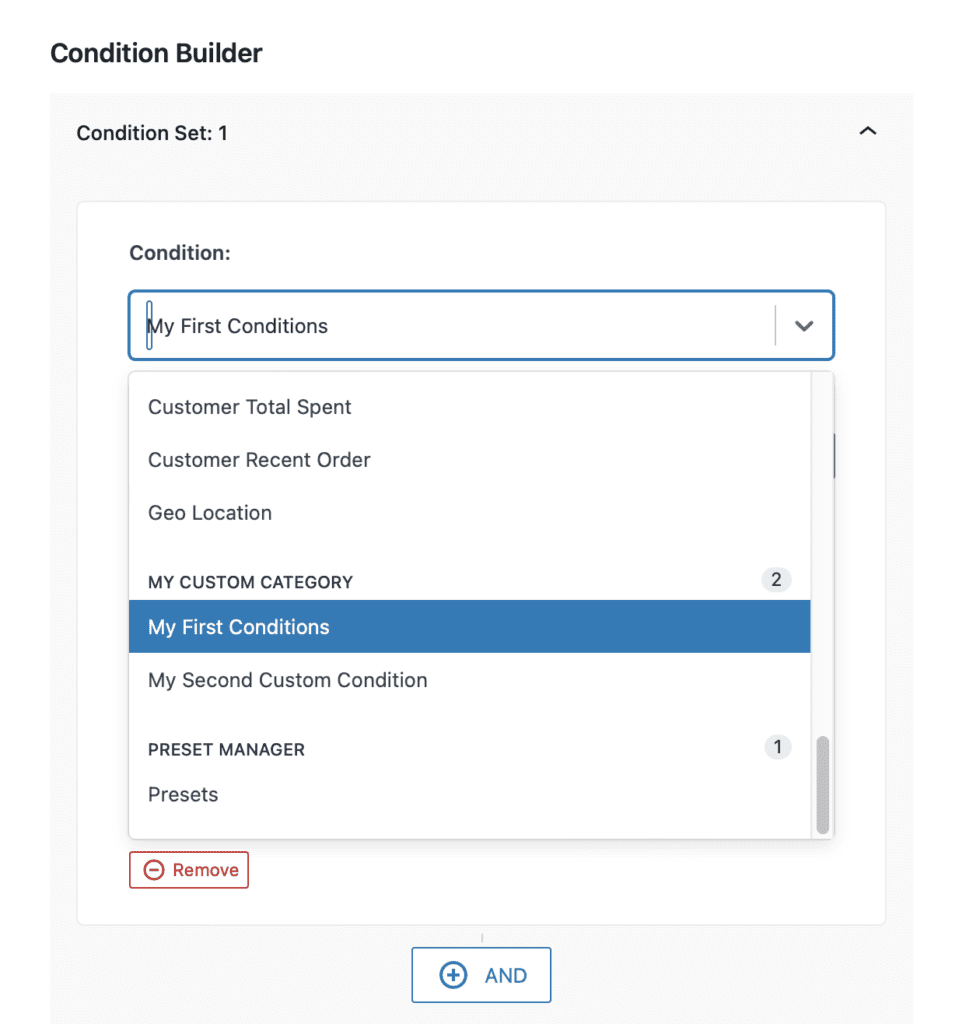
Note: Third-party plugin integrations should always have their own category instead of relying on the built-in categories.
Filter: conditional_blocks_register_condition_categories
Example Snippet
/**
* Register a new category in the Condition Builder.
*/
function example_condition_categories( $categories ) {
$categories[] = [
'value' => 'my_custom_category',
'label' => 'My Custom Category',
];
return $categories;
}
add_filter( 'conditional_blocks_register_condition_categories', 'example_condition_categories', 10, 1 );
Code language: PHP (php)
Register a Condition Type
Custom conditions are registered via a WordPress hook filter.
Each condition requires a unique identifier known as the condition type. The condition type should never change, so choose the name carefully. The configured condition will be saved onto the block’s attribute data, then referenced later when running the condition check.
Available Field Types
You can set up custom fields for users to configure the parameters of each registered condition. These fields will appear once the user select the Condition Type within the Condition Builder.
Ensure that each field key remains the same since it will be used to pass user set value to the Condition Check function.
- Text
- Select
- Number
- Radio
- BlockAction
Need more field types? Let us know.
Filter: conditional_blocks_register_condition_types
Example Snippet
/**
* Register Conditions within group.
*/
function example_conditions( $conditions ) {
// Add a new condition to your group.
$conditions[] = [
'type' => 'my_first_custom_condition', // Important: The type identities the condition and should NOT be changed.
'label' => 'My First Condition',
'description' => 'This condition does something powerful.', // Appears above any fields.
'category' => 'my_custom_category', // A category the condition will appear in.
'fields' => [
[
'key' => 'my_first_option', // Set a unique field key to retrieve later.
'type' => 'text', // Field type.
'attributes' => [
'value' => get_option( 'my_custom_option', 'Default' ), // Set the default value.
'placeholder' => get_option( 'my_custom_option', 'Default' ),
'help' => 'One per line',
],
],
[
'key' => 'my_select', // Set a unique field key to retrieve later.
'type' => 'select', // Field type.
'attributes' => [
'label' => 'Select one of the options', // Label for above the input.
'help' => 'Additional text to help the user', // Help text for under the input
'value' => [
'value' => 'my_second_option',
'label' => 'My second option',
], // Set a default value.
'placeholder' => 'Select an option',
'multiple' => false, // Allow multiple to be selected.
],
'options' => [ // Drop down options for the section. Value & name required.
[
'value' => 'my_first_option',
'label' => 'My first option',
],
[
'value' => 'my_second_option',
'label' => 'My second option',
],
[
'value' => 'my_third_option',
'label' => 'My third option',
],
],
],
[
'key' => 'my_dynamic_field', // Set a unique field key to retrieve later.
'type' => 'text', // Field type.
'requires' => [
'my_first_option' => 'yes',
'my_select' => ['my_second_option', 'my_third_option']
],
'attributes' => [
'value' => get_option( 'my_custom_option', 'Default' ), // Set the default value.
'placeholder' => get_option( 'my_custom_option', 'Default' ),
'help' => 'One per line',
],
],
[
'key' => 'blockAction', // Default key.
'type' => 'blockAction', // Reuse the common field for chosing the Block Action.
],
],
];
// Add a new condition to your group.
$conditions[] = [
'type' => 'my_second_custom_condition', // Give the condition a unique type.
'label' => 'My Second Custom Condition',
'description' => 'This is my second condition with a few different fields.', // Appears above any fields.
'category' => 'my_custom_category', // Group the condition will appear in.
'fields' => [
[
'key' => 'my_number',
'type' => 'number',
'attributes' => [
'label' => 'My Number Field',
'value' => '35',
'placeholder' => '50',
'help' => 'Pick a number between 20-100, with a step of 5.',
'min' => 20,
'max' => 100,
'step' => 5,
],
],
[
'key' => 'my_text',
'type' => 'text',
'attributes' => [
'label' => 'My Text Field',
'help' => 'Useful text or the field',
'value' => get_option( 'custom_value_example', 'Default' ),
'placeholder' => get_option( 'custom_value_example', 'Default' ),
],
],
[
'key' => 'my_radio',
'type' => 'radio', // Field type.
'attributes' => [
'label' => 'Select one of the options', // Label for the input.
'help' => 'Help text for radio',
'value' => 'my_first_option', // Set a default value.
],
'options' => [ // Drop down options for the section. Value & name required.
[
'value' => 'my_first_option',
'label' => 'My first option',
],
[
'value' => 'my_second_option',
'label' => 'My second option',
],
],
],
],
];
return $conditions;
}
add_filter( 'conditional_blocks_register_condition_types', 'example_conditions', 10, 1 );
Code language: PHP (php)
Register a Condition Check
Each Condition Type requires its own Condition Check.
The Condition Check is triggered when WordPress block is about to be rendered on the server-side. The purpose of the Condition Check is to determine whether the block should be rendered for the current visitors.
The Condition Fields configured by the user are accessible using the $condition
object. The values set for each field should be accessed using the field key from above.
$condition['my_text']; // The value written into the text field by the user.
Code language: PHP (php)
Filter: conditional_blocks_register_check_WRITE_CONDITION_TYPE_HERE
Example Snippet
/**
* Check for the Condition Type: my_first_custom_condition
*
* NOTE: defaults are not translated from above if the user doesn't interact with the React fields.
*
* @param bool $should_render if condition passed validation.
* @param array $condition contains the configured conditions with keys/values.
* @return bool $should_block_render - defaults to false.
*/
function example_my_first_custom_check( $should_block_render, $condition ) {
$has_match = false;
// Check something with the user set criteria, the block has a match if the my_text field has "yes" as the value.
if ( ! empty( $condition['my_text'] ) && $condition['my_text'] === 'yes' ) {
$has_match = true;
}
// Reuse the Block Action from other conditions.
$block_action = ! empty( $condition['blockAction'] ) ? $condition['blockAction'] : 'showBlock';
if ( $has_match && $block_action === 'showBlock' ) {
$should_block_render = true;
} elseif ( ! $has_match && $block_action === 'hideBlock' ) {
$should_block_render = true;
}
return $should_block_render;
}
add_filter( 'conditional_blocks_register_check_my_first_custom_condition', 'example_my_first_custom_check', 10, 2 );
Code language: PHP (php)
Share your conditions!
Congratulations! If you’ve made it this far, then you’ve successfully created your own visibility conditions for WordPress content.
We’d love to feature what you’ve made! 🥳 🦾